Introduction
Think about having the complete potential of your favourite AI assistant, reworking it from a useful device right into a inventive powerhouse. Image a world the place your interactions with Massive Language Fashions(LLMs) are environment friendly, profoundly intuitive, and impactful. Enter the Skeleton of Ideas (SoT) – a groundbreaking framework poised to revolutionize synthetic intelligence and pure language processing. Let’s look at this intriguing concept in additional element and see the way it would possibly enhance your AI-powered inventive and problem-solving processes.
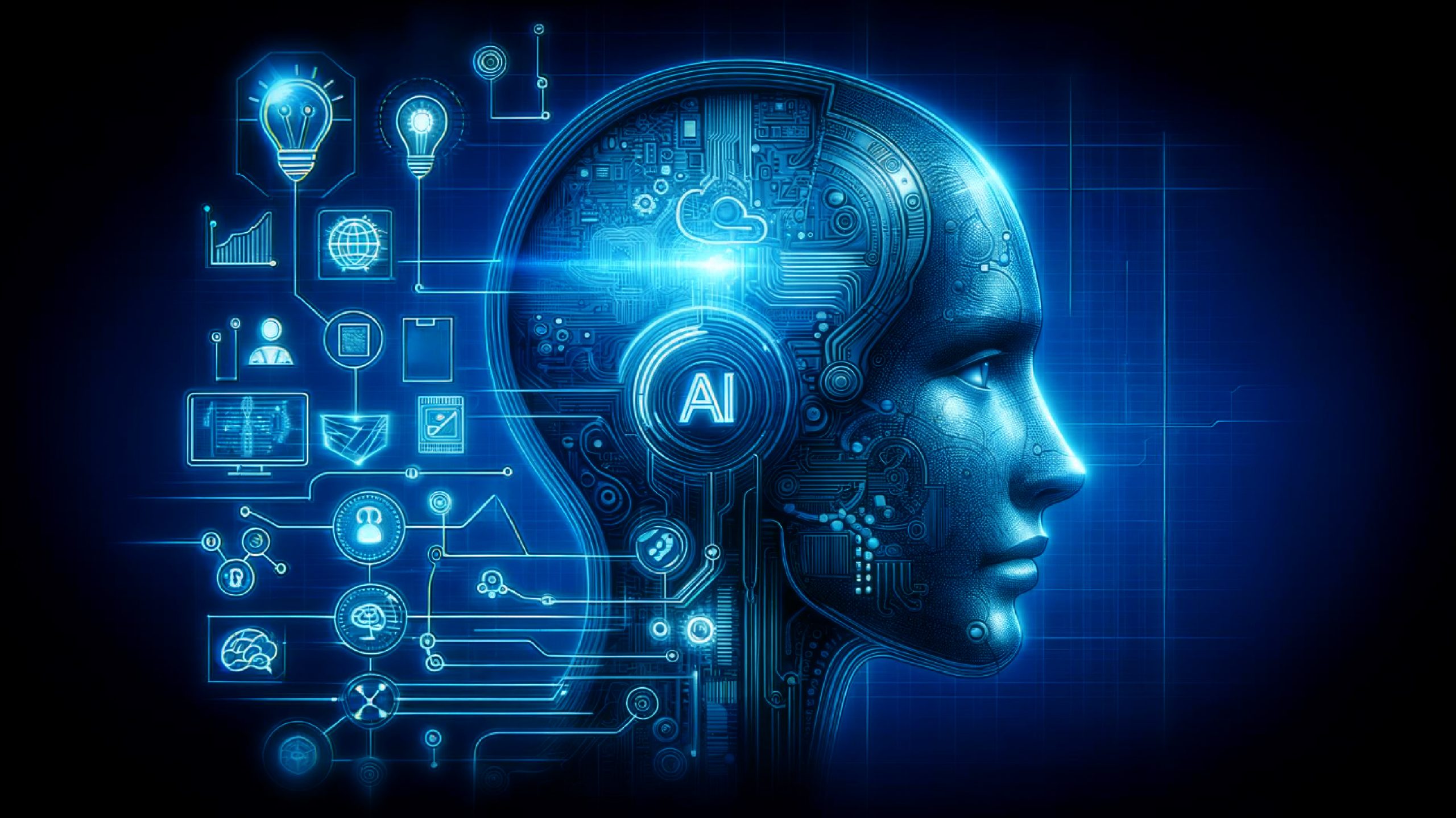
Overview
- The Skeleton of Ideas (SoT) framework transforms AI right into a inventive powerhouse with structured but adaptable outlines for complicated duties.
- SoT allows AI to handle challenges with readability and depth by offering a versatile conceptual framework.
- Core components of SoT embrace structured outlines, versatile enlargement, hierarchical considering, iterative growth, and cross-linking.
- Implementing SoT includes making a skeletal define, increasing factors, and connecting elements utilizing OpenAI’s GPT mannequin.
- SoT affords readability, scalability, flexibility, holistic understanding, and environment friendly exploration of complicated matters.
- Actual-world purposes of SoT embrace tutorial analysis, product growth, and strategic planning for structured exploration of intricate topics.
Revealing the Thought Skeleton
What if you happen to might assemble an AI conceptual framework that permits flexibility and creativity whereas guiding the mannequin’s thought course of? That’s exactly the purpose of the Skeleton of Ideas. SoT allows AI fashions to sort out challenges with unprecedented readability, coherence, and depth by offering a structured but adaptable define for complicated duties.
The Core Idea of SoT
- Structured Define: A high-level construction outlining the primary components of the project.
- Versatile Enlargement: The entire skeletal parts could be improved and expanded upon as required.
- Hierarchical Pondering: Concepts are organized in a logical, nested construction.
- Iterative Growth: The skeleton modifications because the AI investigates and refines ideas.
- Cross-Linking: Dynamically created connections between varied skeleton parts are potential.
Implementing the Skeleton of Ideas
Let’s deliver this idea to life with a Python implementation that leverages OpenAI’s GPT mannequin:
Pre-Requisite and Setup
!pip set up openai --upgrade
Importing Libraries
from openai importOpenAI
import openai
import time
import re
from IPython.show import Markdown, show
Setting API Key Configuration
os.environ["OPENAI_API_KEY"]= “Your openAPIKey”
from openai import OpenAI
from openai import OpenAI
import time
import re
class SkeletonOfThoughts:
"""
A category to create and manipulate a skeletal define utilizing OpenAI's API.
"""
def __init__(self, api_key, mannequin="gpt-3.5-turbo"):
"""
Initialize the SkeletonOfThoughts object.
Args:
api_key (str): OpenAI API key
mannequin (str): OpenAI mannequin to make use of (default is "gpt-3.5-turbo")
"""
self.shopper = OpenAI(api_key=api_key)
self.mannequin = mannequin
self.skeleton = {}
def create_skeleton(self, subject):
"""
Create a skeletal define for the given subject.
subject (str): The subject to create a skeleton for
Returns:
dict: The created skeleton define
"""
immediate = f"""Create an in depth skeletal define for the subject: '{subject}'.
Use the next format:
1. Major level
1.1. Subpoint
1.2. Subpoint
2. Major level
2.1. Subpoint
2.2. Subpoint
Present not less than 3 details with 2-3 subpoints every."""
attempt:
response = self.execute_prompt(immediate, max_tokens=500)
print(f"Uncooked API Response:n{response}") # Debug print
self.skeleton = self.parse_skeleton(response)
print(f"Parsed Skeleton:n{self.skeleton}") # Debug print
return self.skeleton
besides Exception as e:
print(f"Error creating skeleton: {str(e)}")
return {}
def expand_point(self, point_number):
"""
Increase a selected level within the skeleton.
point_number (int): The variety of the purpose to develop
Returns:
str: The expanded level content material
"""
point_key = self.find_point_key(point_number)
if point_key is None:
return f"Level {point_number} not discovered within the skeleton."
current_content = self.skeleton[point_key]
immediate = f"Increase on the next level: {current_content}"
enlargement = self.execute_prompt(immediate)
self.skeleton[point_key] = f"{current_content}n{enlargement}"
return self.skeleton[point_key]
def add_cross_link(self, point1, point2):
"""
Add a cross-link between two factors within the skeleton.
point1 (int): The variety of the primary level
point2 (int): The variety of the second level
Returns:
str: The created cross-link rationalization
"""
key1 = self.find_point_key(point1)
key2 = self.find_point_key(point2)
if key1 is None or key2 is None:
return "One or each factors not discovered within the skeleton."
immediate = f"Clarify how these two factors are associated: 1) {self.skeleton[key1]} 2) {self.skeleton[key2]}"
hyperlink = self.execute_prompt(immediate)
link_key = f"Hyperlink: {key1} - {key2}"
self.skeleton[link_key] = hyperlink
return hyperlink
def execute_prompt(self, immediate, max_tokens=500):
"""
Execute a immediate utilizing the OpenAI API.
immediate (str): The immediate to ship to the API
max_tokens (int): Most variety of tokens within the response
Returns:
str: The API response content material
"""
attempt:
response = self.shopper.chat.completions.create(
mannequin=self.mannequin,
messages=[
{"role": "system", "content": "You are an AI assistant creating a structured outline."},
{"role": "user", "content": prompt}
],
max_tokens=max_tokens
)
content material = response.selections[0].message.content material.strip()
print(f"API Response Content material:n{content material}") # Debug print
return content material
besides Exception as e:
print(f"Error executing immediate: {str(e)}")
return ""
def parse_skeleton(self, textual content):
"""
Parse the skeleton textual content right into a structured dictionary.
textual content (str): The uncooked skeleton textual content to parse
Returns:
dict: The parsed skeleton construction
"""
print("Parsing textual content:", textual content) # Debug print
traces = textual content.cut up('n')
skeleton = {}
current_main_point = ""
for line in traces:
line = line.strip()
if not line:
proceed
# Match details (1., 2., and so on.)
main_point_match = re.match(r'^(d+.)s*(.*)', line)
if main_point_match:
current_main_point = main_point_match.group(1)
skeleton[current_main_point] = main_point_match.group(2)
# Match subpoints (1.1., 1.2., 2.1., and so on.)
subpoint_match = re.match(r'^(d+.d+.)s*(.*)', line)
if subpoint_match:
subpoint_key = subpoint_match.group(1)
skeleton[subpoint_key] = subpoint_match.group(2)
# If it isn't a numbered level, add it to the present fundamental level
elif current_main_point and line:
skeleton[current_main_point] += " " + line
return skeleton
def find_point_key(self, point_number):
"""
Discover the important thing within the skeleton dictionary for a given level quantity.
point_number (int): The purpose quantity to search out
Returns:
str: The important thing within the skeleton dictionary, or None if not discovered
"""
for key in self.skeleton.keys():
if key.startswith(str(point_number) + '.'):
return key
return None
def display_skeleton(self):
"""
Show the present skeleton construction.
"""
if not self.skeleton:
print("The skeleton is empty.")
else:
for key, worth in self.skeleton.gadgets():
print(f"{key} {worth}n")
def fundamental():
"""
Major operate to show the utilization of SkeletonOfThoughts class.
"""
api_key = key # Substitute together with your precise OpenAI API key
if api_key == "your-api-key-here":
print("Please exchange 'your-api-key-here' together with your precise OpenAI API key.")
return
attempt:
sot = SkeletonOfThoughts(api_key)
subject = "The Influence of Synthetic Intelligence on Future Job Markets"
print(f"Creating skeleton for subject: {subject}")
initial_skeleton = sot.create_skeleton(subject)
if not initial_skeleton:
print("Did not create preliminary skeleton. Please examine the error messages above.")
return
print("nInitial Skeleton:")
sot.display_skeleton()
# Increase a degree
print("nExpanding Level 1:")
expanded_point = sot.expand_point(1)
print(expanded_point)
# Add a cross-link
print("nAdding Cross-link between factors 1 and a couple of:")
hyperlink = sot.add_cross_link(1, 2)
print(hyperlink)
print("nFinal Skeleton:")
sot.display_skeleton()
besides Exception as e:
print(f"An error occurred: {str(e)}")
if __name__ == "__main__":
fundamental()
Output With Rationalization
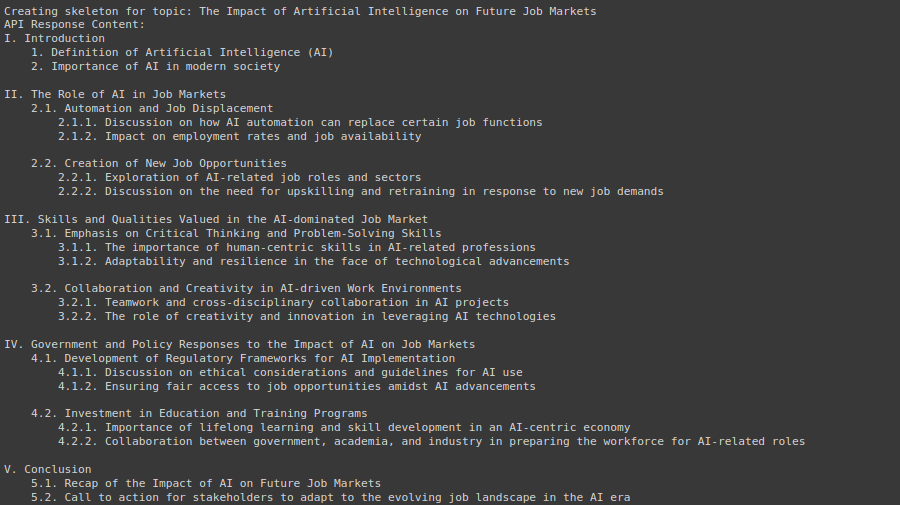
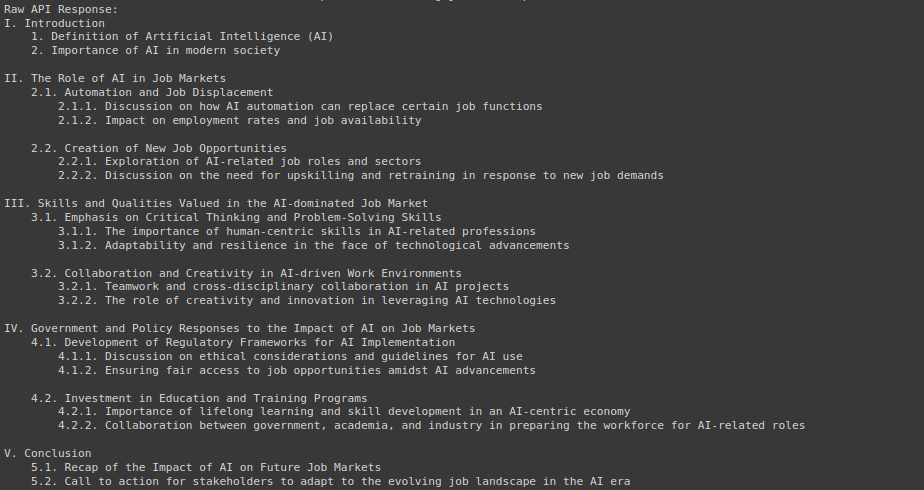
Creating skeleton: The system created an in depth define for the subject “The Influence of Synthetic Intelligence on Future Job Markets.” This define consists of details and subpoints protecting varied elements of AI’s impression on job markets.
Preliminary Skeleton: The parsed skeleton is displayed, displaying the define’s construction with details and subpoints. Nonetheless, there appear to be some parsing points, as some subpoints are incorrectly related to details.
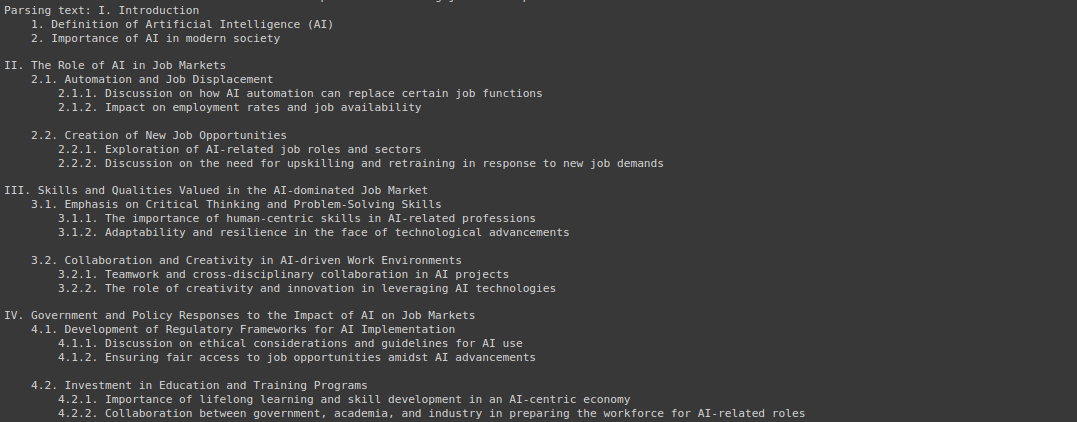
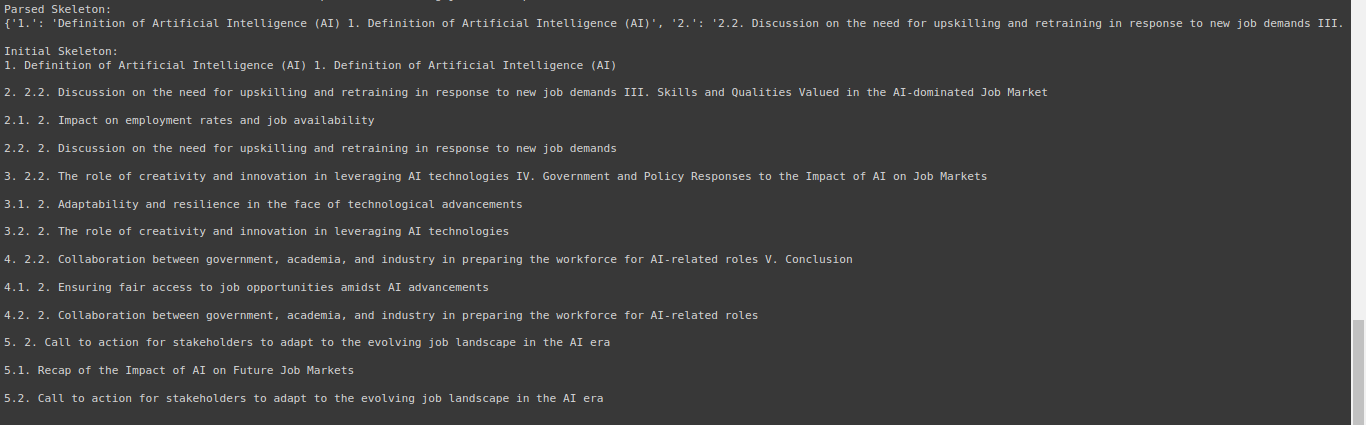
Increasing Level 1: The system expanded on the primary level, offering an in depth rationalization of the definition of Synthetic Intelligence. This enlargement consists of what AI is, its parts, potential impacts, and moral issues.
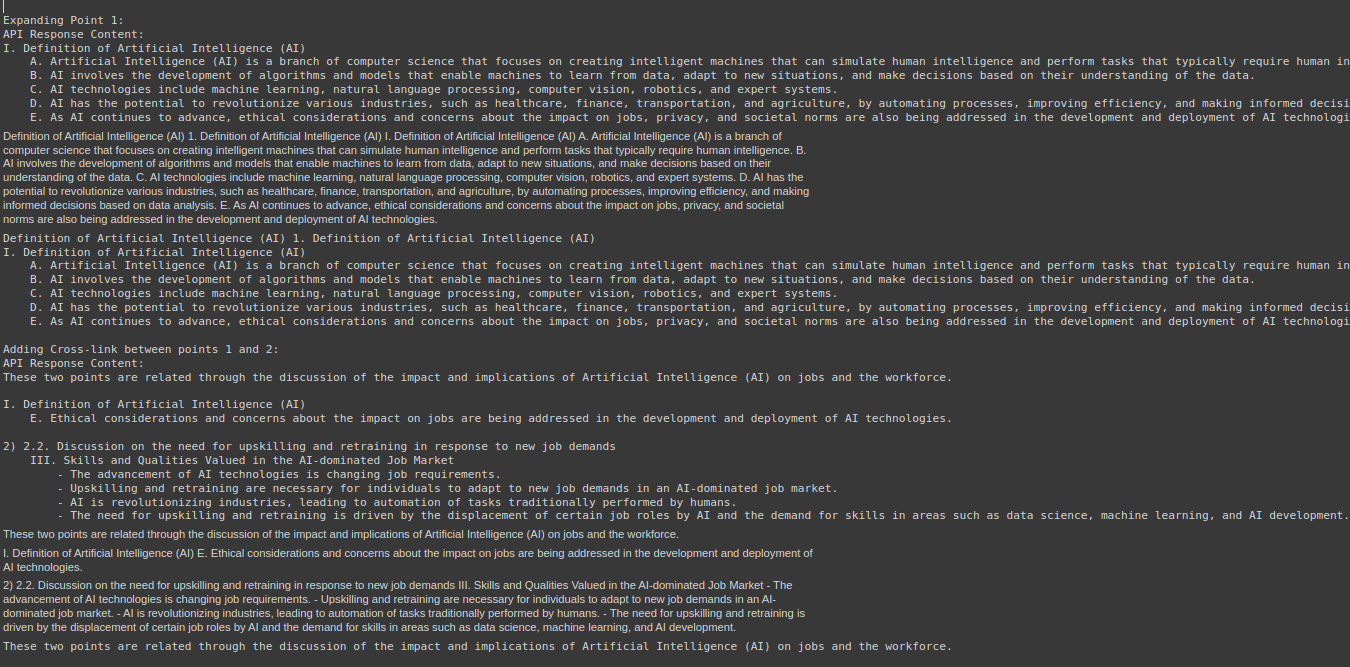
Including Cross-link between factors 1 and a couple of: The system linked the primary level (Definition of AI) and the second level (Dialogue on upskilling and retraining). It explains how these factors are associated by means of their shared concentrate on AI’s impression on jobs and the workforce.
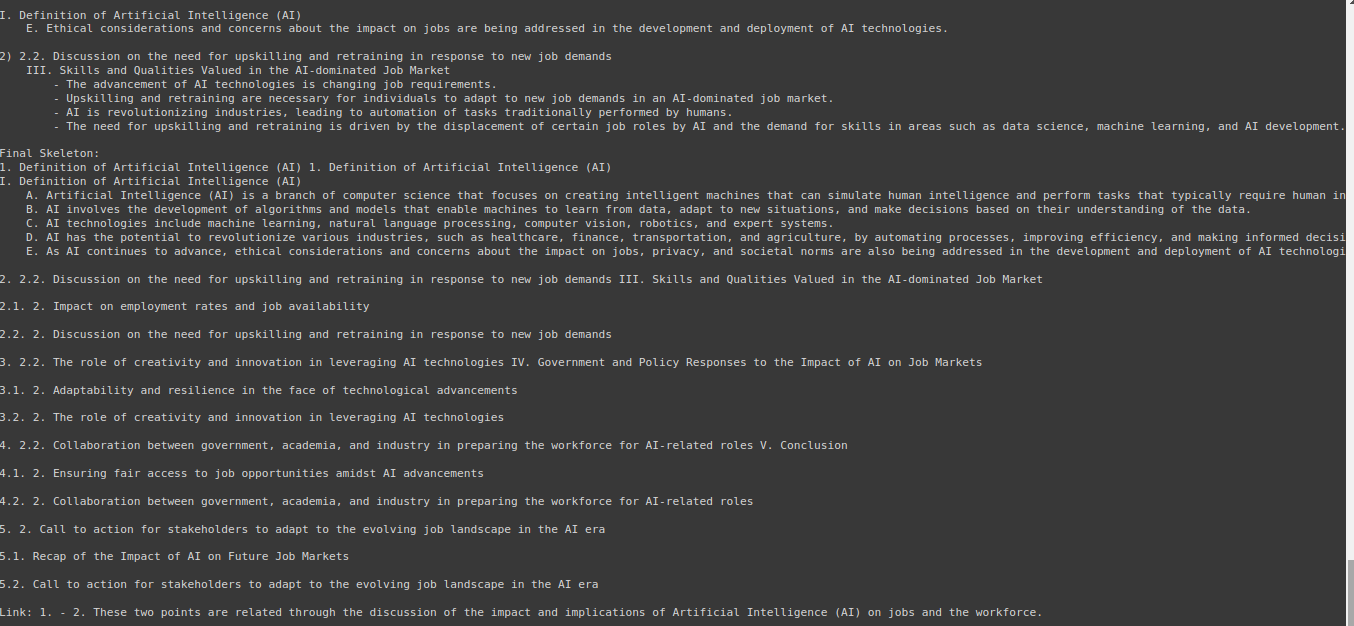

Last Skeleton: The ultimate skeleton combines all of the earlier steps. It consists of the preliminary define, the expanded first level, and the cross-link between factors 1 and a couple of. This supplies a complete construction for discussing AI’s impression on future job markets.
This implementation brings the Skeleton of Ideas to life:
- Now we have created a Skeleton Of Ideas class that encapsulates our method, initialized with an OpenAI API key and a mannequin alternative (defaulting to “gpt3.5-turbo”).
- The create_skeleton technique generates an preliminary define for a given subject:
- It makes use of a selected immediate format to make sure a structured response from the AI.
- The strategy handles API interactions and parses the response right into a dictionary construction.
- expand_point permits for deeper exploration of particular factors within the skeleton:
- It identifies the purpose to develop based mostly on its quantity.
- The strategy then generates extra content material for that time utilizing the AI.
- add_cross_link establishes connections between totally different elements of the skeleton:
- It takes two-point numbers as enter and explains their relationship.
- The hyperlink is then added to the skeleton as a brand new entry.
- The skeleton is saved as a dictionary, with keys representing level numbers (e.g., “1.”, “1.1.”) and values containing the content material.
- Extra helper strategies improve performance:
- execute_prompt handles API calls with error administration and debugging output.
- parse_skeleton converts the AI’s textual content response right into a structured dictionary.
- find_point_key locates particular factors within the skeleton.
- display_skeleton prints the present state of the skeleton.
The Magic of SoT in Motion
Let’s break down what occurs after we run this code:
- Preliminary Skeleton Creation
- The AI generates a high-level define for the given subject (e.g., “The Influence of AI on Future Job Markets”).
- This define consists of details and subpoints, formatted as a numbered checklist.
- Level Enlargement
- We will dive deeper into particular factors (e.g., level 1) utilizing the expand_point technique.
- The AI supplies extra detailed details about the chosen level.
- CrossLinking
- Connections between totally different elements of the skeleton are established utilizing add_cross_link.
- For instance, we are able to hyperlink factors 1 and a couple of, producing an evidence of their relationship.
- Iterative Growth
- The skeleton could be repeatedly refined by increasing factors or including crosslinks.
- Every operation updates the skeleton dictionary, permitting for cumulative information constructing.
- Sturdy Error Dealing with
- The implementation consists of intensive error checking and debugging output.
- This helps establish and tackle points with API calls or parsing.
This structured but versatile method permits for a extra complete and interconnected exploration of complicated matters. Utilizing AI to generate content material and the programmatic construction for organizing and linking concepts creates a robust device for thought growth and evaluation.
Additionally, Learn these Immediate Engineering articles:
Article | Supply |
Implementing the Tree of Ideas Technique in AI | Hyperlink |
What are Delimiters in Immediate Engineering? | Hyperlink |
What’s Self-Consistency in Immediate Engineering? | Hyperlink |
What’s Temperature in Immediate Engineering? | Hyperlink |
Test extra articles right here – Immediate Engineering.
Benefits of the Skeleton of Ideas
Listed here are the benefits of the skeleton of ideas:
- Readability and Group: Concepts are structured in a logical, easy-to-follow method.
- Scalability: The skeleton could be as easy or as complicated as the duty requires.
- Flexibility: Straightforward to adapt and refine as new insights emerge.
- Holistic Understanding: Crosslinking promotes a extra complete grasp of the subject.
- Environment friendly Exploration: Supplies a roadmap for thorough investigation with out getting misplaced in particulars.
Actual World Functions
Listed here are the real-world purposes:
- Educational Analysis: Think about utilizing SoT to stipulate a posh analysis paper. The skeleton might present the primary sections and subsections, with the flexibility to develop on key factors and set up connections between totally different elements of the argument.
- Product Growth: Within the tech trade, SoT could possibly be used to map out the options and parts of a brand new product, permitting groups to visualise the general construction whereas diving into particular particulars as wanted.
- Strategic Planning: Companies might use SoT to develop complete methods, outlining fundamental goals, potential challenges, and motion plans, with the pliability to develop and join totally different elements of the technique.
Challenges and Issues
Whereas the Skeleton of Ideas affords thrilling prospects, it’s vital to contemplate:
- Balancing Construction and Flexibility: Discovering the correct stability between offering a transparent construction and permitting for inventive exploration.
- Managing Complexity: Managing the skeleton’s development and interconnections can turn out to be difficult for very giant or intricate matters.
- AI Limitations: The skeleton’s high quality and expansions are nonetheless certain by the capabilities of the underlying language mannequin.
The Way forward for Immediate Engineering
As AI advances, methods just like the Skeleton of Ideas will improve our capacity to sort out complicated issues and discover intricate matters. Offering a structured but versatile framework for AI-assisted considering opens up new prospects for information group, problem-solving, and artistic exploration.
Conclusion
The Skeleton of Ideas represents a major step in structuring and guiding AI-assisted thought processes. By offering a versatile scaffolding for concepts, we are able to discover complicated matters with unprecedented readability and depth whereas sustaining the flexibility to adapt and refine our method as new insights emerge.
Whether or not you’re a researcher, a enterprise strategist, or somebody who loves exploring concepts, the Skeleton of Ideas method affords a robust new device for organizing and growing your ideas. So why not give it a attempt? You would possibly simply uncover a brand new mind-set that transforms the way you method complicated matters and challenges!
Regularly Requested Questions
Ans. The Skeleton of Ideas is a immediate engineering method that gives a structured define or framework for an AI mannequin to comply with when producing responses. It breaks down complicated duties right into a logical sequence of steps or parts, guiding the AI’s thought course of with out totally specifying every step’s content material. This method helps preserve coherence and construction in longer or extra complicated outputs.
Ans. Whereas each methods intention to information AI reasoning, the Skeleton of Ideas supplies a higher-level construction or define, leaving extra room for the AI to fill in particulars. The Algorithm of Ideas, then again, usually specifies extra detailed step-by-step directions. The Skeleton method is commonly extra versatile and helpful for inventive or open-ended duties.
Ans. A number of “ideas” methods have been developed in immediate engineering to boost AI reasoning and output high quality. Some notable ones embrace:
a) Tree of Ideas: This technique creates a tree-like construction of potential reasoning paths, permitting the AI to discover a number of traces of thought and choose essentially the most promising one.
b) Chain of Thought: This system encourages the AI to indicate its step-by-step reasoning course of, bettering transparency and permitting for extra complicated problem-solving.
c) Graph of Ideas: That is much like the Tree of Ideas however permits for extra complicated interconnections between concepts. It’s helpful for duties requiring non-linear considering.
d) Reflexion: This method includes the AI critiquing and refining its outputs, resulting in improved accuracy and coherence.
e) ReAct: Combines reasoning and performing, permitting the AI to iteratively cause a couple of activity and take actions based mostly on that reasoning.