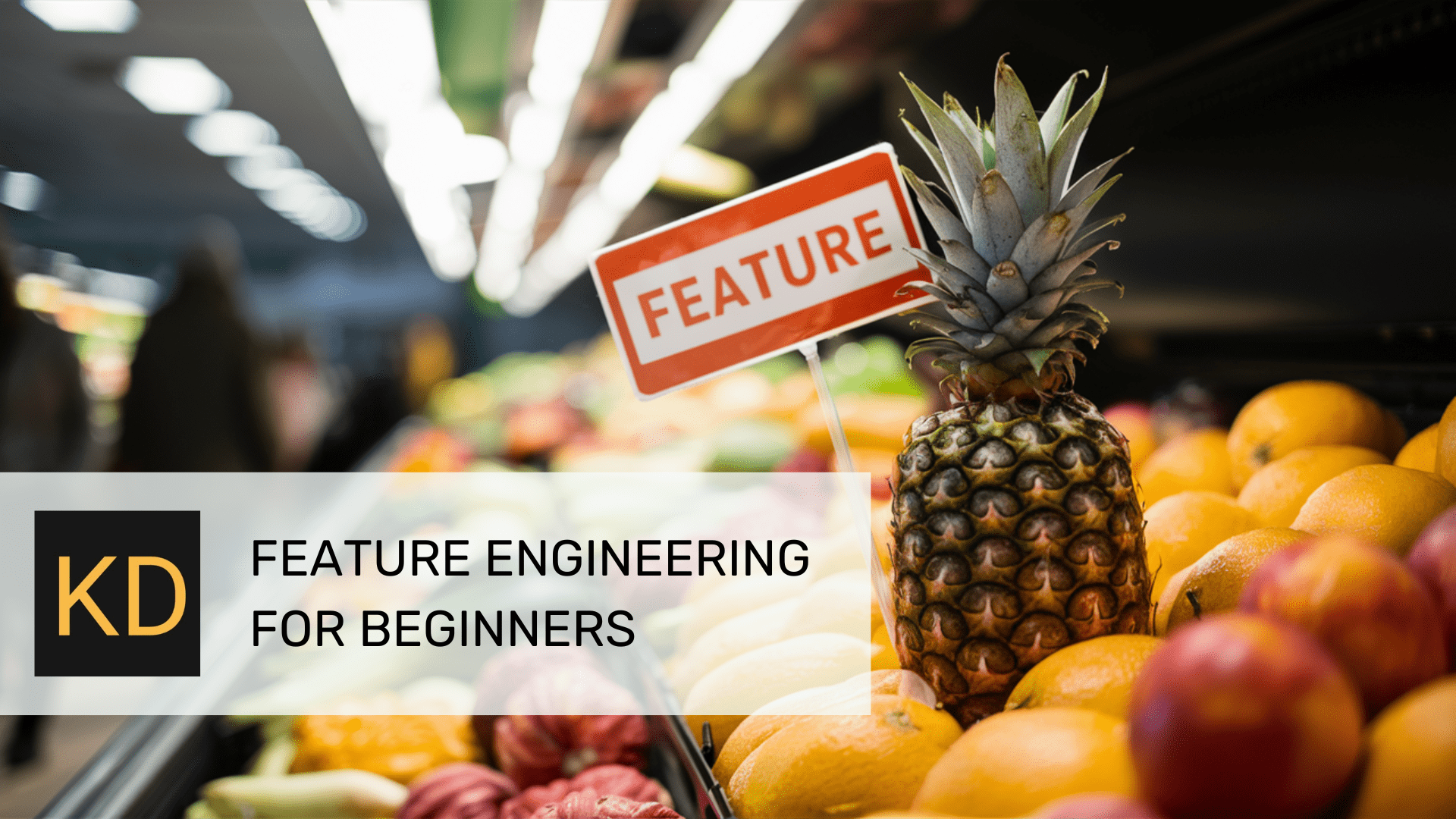
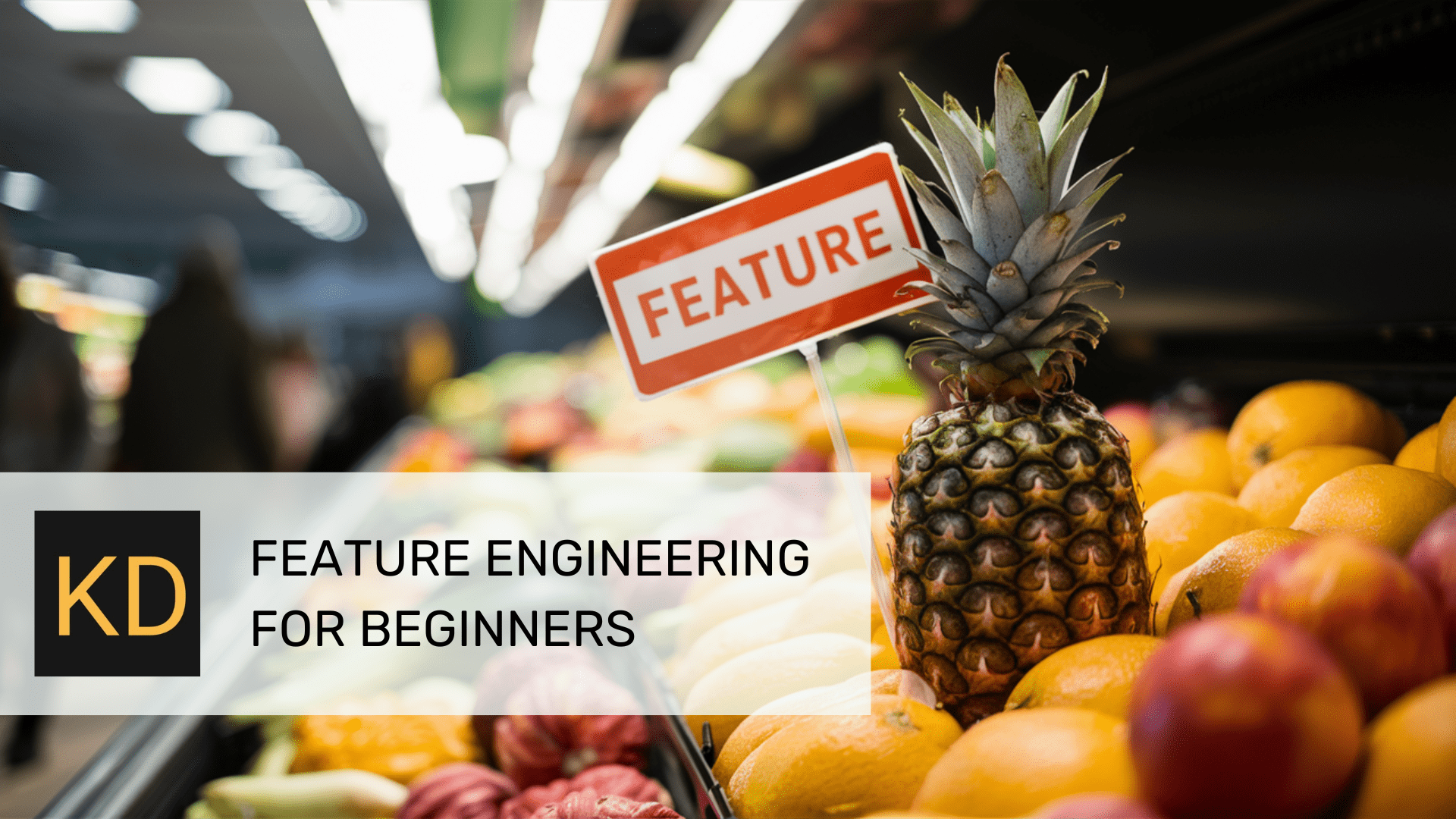
Picture created by Creator
Introduction
Function engineering is among the most vital elements of the machine studying pipeline. It’s the follow of making and modifying options, or variables, for the needs of bettering mannequin efficiency. Effectively-designed options can rework weak fashions into sturdy ones, and it’s by way of characteristic engineering that fashions can change into each extra strong and correct. Function engineering acts because the bridge between the dataset and the mannequin, giving the mannequin the whole lot it must successfully clear up an issue.
It is a information supposed for brand spanking new knowledge scientists, knowledge engineers, and machine studying practitioners. The target of this text is to speak elementary characteristic engineering ideas and supply a toolbox of methods that may be utilized to real-world eventualities. My purpose is that, by the top of this text, you may be armed with sufficient working data about characteristic engineering to use it to your personal datasets to be fully-equipped to start creating highly effective machine studying fashions.
Understanding Options
Options are measurable traits of any phenomenon that we’re observing. They’re the granular components that make up the info with which fashions function upon to make predictions. Examples of options can embrace issues like age, earnings, a timestamp, longitude, worth, and nearly anything one can consider that may be measured or represented in some type.
There are completely different characteristic sorts, the principle ones being:
- Numerical Options: Steady or discrete numeric sorts (e.g. age, wage)
- Categorical Options: Qualitative values representing classes (e.g. gender, shoe measurement sort)
- Textual content Options: Phrases or strings of phrases (e.g. “this” or “that” or “even this”)
- Time Sequence Options: Knowledge that’s ordered by time (e.g. inventory costs)
Options are essential in machine studying as a result of they instantly affect a mannequin’s capacity to make predictions. Effectively-constructed options enhance mannequin efficiency, whereas dangerous options make it tougher for a mannequin to supply sturdy predictions. Function choice and have engineering are preprocessing steps within the machine studying course of which might be used to organize the info to be used by studying algorithms.
A distinction is made between characteristic choice and have engineering, although each are essential in their very own proper:
- Function Choice: The culling of vital options from the complete set of all out there options, thus decreasing dimensionality and selling mannequin efficiency
- Function Engineering: The creation of recent options and subsequent altering of current ones, all in assistance from making a mannequin carry out higher
By deciding on solely an important options, characteristic choice helps to solely go away behind the sign within the knowledge, whereas characteristic engineering creates new options that assist to mannequin the result higher.
Primary Methods in Function Engineering
Whereas there are a variety of primary characteristic engineering methods at our disposal, we’ll stroll by way of a few of the extra vital and well-used of those.
Dealing with Lacking Values
It is not uncommon for datasets to comprise lacking data. This may be detrimental to a mannequin’s efficiency, which is why you will need to implement methods for coping with lacking knowledge. There are a handful of frequent strategies for rectifying this concern:
- Imply/Median Imputation: Filling lacking areas in a dataset with the imply or median of the column
- Mode Imputation: Filling lacking spots in a dataset with the commonest entry in the identical column
- Interpolation: Filling in lacking knowledge with values of information factors round it
These fill-in strategies must be utilized based mostly on the character of the info and the potential impact that the tactic might need on the top mannequin.
Coping with lacking data is essential in holding the integrity of the dataset in tact. Right here is an instance Python code snippet that demonstrates numerous knowledge filling strategies utilizing the pandas
library.
import pandas as pd
from sklearn.impute import SimpleImputer
# Pattern DataFrame
knowledge = {'age': [25, 30, np.nan, 35, 40], 'wage': [50000, 60000, 55000, np.nan, 65000]}
df = pd.DataFrame(knowledge)
# Fill in lacking ages utilizing the imply
mean_imputer = SimpleImputer(technique='imply')
df['age'] = mean_imputer.fit_transform(df[['age']])
# Fill within the lacking salaries utilizing the median
median_imputer = SimpleImputer(technique='median')
df['salary'] = median_imputer.fit_transform(df[['salary']])
print(df)
Encoding of Categorical Variables
Recalling that almost all machine studying algorithms are finest (or solely) outfitted to take care of numeric knowledge, categorical variables should typically be mapped to numerical values to ensure that stated algorithms to raised interpret them. The most typical encoding schemes are the next:
- One-Sizzling Encoding: Producing separate columns for every class
- Label Encoding: Assigning an integer to every class
- Goal Encoding: Encoding classes by their particular person end result variable averages
The encoding of categorical knowledge is critical for planting the seeds of understanding in lots of machine studying fashions. The correct encoding technique is one thing you’ll choose based mostly on the precise state of affairs, together with each the algorithm at use and the dataset.
Beneath is an instance Python script for the encoding of categorical options utilizing pandas
and components of scikit-learn
.
import pandas as pd
from sklearn.preprocessing import OneHotEncoder, LabelEncoder
# Pattern DataFrame
knowledge = {'shade': ['red', 'blue', 'green', 'blue', 'red']}
df = pd.DataFrame(knowledge)
# Implementing one-hot encoding
one_hot_encoder = OneHotEncoder()
one_hot_encoding = one_hot_encoder.fit_transform(df[['color']]).toarray()
df_one_hot = pd.DataFrame(one_hot_encoding, columns=one_hot_encoder.get_feature_names(['color']))
# Implementing label encoding
label_encoder = LabelEncoder()
df['color_label'] = label_encoder.fit_transform(df['color'])
print(df)
print(df_one_hot)
Scaling and Normalizing Knowledge
For good efficiency of many machine studying strategies, scaling and normalization must be carried out in your knowledge. There are a number of strategies for scaling and normalizing knowledge, comparable to:
- Standardization: Reworking knowledge in order that it has a imply of 0 and a typical deviation of 1
- Min-Max Scaling: Scaling knowledge to a set vary, comparable to [0, 1]
- Sturdy Scaling: Scaling excessive and low values iteratively by the median and interquartile vary, respectively
The scaling and normalization of information is essential for making certain that characteristic contributions are equitable. These strategies enable the various characteristic values to contribute to a mannequin commensurately.
Beneath is an implementation, utilizing scikit-learn
, that reveals the right way to full knowledge that has been scaled and normalized.
import pandas as pd
from sklearn.preprocessing import StandardScaler, MinMaxScaler, RobustScaler
# Pattern DataFrame
knowledge = {'age': [25, 30, 35, 40, 45], 'wage': [50000, 60000, 55000, 65000, 70000]}
df = pd.DataFrame(knowledge)
# Standardize knowledge
scaler_standard = StandardScaler()
df['age_standard'] = scaler_standard.fit_transform(df[['age']])
# Min-Max Scaling
scaler_minmax = MinMaxScaler()
df['salary_minmax'] = scaler_minmax.fit_transform(df[['salary']])
# Sturdy Scaling
scaler_robust = RobustScaler()
df['salary_robust'] = scaler_robust.fit_transform(df[['salary']])
print(df)
The fundamental methods above together with the corresponding instance code present pragmatic options for lacking knowledge, encoding categorical variables, and scaling and normalizing knowledge utilizing powerhouse Python instruments pandas
and scikit-learn
. These methods will be built-in into your personal characteristic engineering course of to enhance your machine studying fashions.
Superior Methods in Function Engineering
We now flip our consideration to to extra superior featured engineering methods, and embrace some pattern Python code for implementing these ideas.
Function Creation
With characteristic creation, new options are generated or modified to style a mannequin with higher efficiency. Some methods for creating new options embrace:
- Polynomial Options: Creation of higher-order options with current options to seize extra advanced relationships
- Interplay Phrases: Options generated by combining a number of options to derive interactions between them
- Area-Particular Function Era: Options designed based mostly on the intricacies of topics inside the given downside realm
Creating new options with tailored that means can tremendously assist to spice up mannequin efficiency. The subsequent script showcases how characteristic engineering can be utilized to convey latent relationships in knowledge to mild.
import pandas as pd
import numpy as np
# Pattern DataFrame
knowledge = {'x1': [1, 2, 3, 4, 5], 'x2': [10, 20, 30, 40, 50]}
df = pd.DataFrame(knowledge)
# Polynomial Options
df['x1_squared'] = df['x1'] ** 2
df['x1_x2_interaction'] = df['x1'] * df['x2']
print(df)
Dimensionality Discount
In an effort to simplify fashions and improve their efficiency, it may be helpful to downsize the variety of mannequin options. Dimensionality discount methods that may assist obtain this objective embrace:
- PCA (Principal Part Evaluation): Transformation of predictors into a brand new characteristic set comprised of linearly unbiased mannequin options
- t-SNE (t-Distributed Stochastic Neighbor Embedding): Dimension discount that’s used for visualization functions
- LDA (Linear Discriminant Evaluation): Discovering new mixtures of mannequin options which might be efficient for deconstructing completely different courses
In an effort to shrink the scale of your dataset and preserve its relevancy, dimensional discount methods will assist. These methods have been devised to deal with the high-dimensional points associated to knowledge, comparable to overfitting and computational demand.
An indication of information shrinking applied with scikit-learn
is proven subsequent.
import pandas as pd
from sklearn.decomposition import PCA
# Pattern DataFrame
knowledge = {'feature1': [2.5, 0.5, 2.2, 1.9, 3.1], 'feature2': [2.4, 0.7, 2.9, 2.2, 3.0]}
df = pd.DataFrame(knowledge)
# Use PCA for Dimensionality Discount
pca = PCA(n_components=1)
df_pca = pca.fit_transform(df)
df_pca = pd.DataFrame(df_pca, columns=['principal_component'])
print(df_pca)
Time Sequence Function Engineering
With time-based datasets, particular characteristic engineering methods have to be used, comparable to:
- Lag Options: Former knowledge factors are used to derive mannequin predictive options
- Rolling Statistics: Knowledge statistics are calculated throughout knowledge home windows, comparable to rolling means
- Seasonal Decomposition: Knowledge is partitioned into sign, development, and random noise classes
Temporal fashions want various augmentation in comparison with direct mannequin becoming. These strategies comply with temporal dependence and patterns to make the predictive mannequin sharper.
An indication of time sequence options augmenting utilized utilizing pandas
is proven subsequent as properly.
import pandas as pd
import numpy as np
# Pattern DataFrame
date_rng = pd.date_range(begin="1/1/2022", finish='1/10/2022', freq='D')
knowledge = {'date': date_rng, 'worth': [100, 110, 105, 115, 120, 125, 130, 135, 140, 145]}
df = pd.DataFrame(knowledge)
df.set_index('date', inplace=True)
# Lag Options
df['value_lag1'] = df['value'].shift(1)
# Rolling Statistics
df['value_rolling_mean'] = df['value'].rolling(window=3).imply()
print(df)
The above examples show sensible functions of superior characteristic engineering methods, by way of utilization of pandas
and scikit-learn
. By using these strategies you may improve the predictive energy of your mannequin.
Sensible Suggestions and Greatest Practices
Listed here are a number of easy however vital suggestions to remember whereas working by way of your characteristic engineering course of.
- Iteration: Function engineering is a trial-and-error course of, and you’ll get higher with it every time you iterate. Take a look at completely different characteristic engineering concepts to search out one of the best set of options.
- Area Information: Make the most of experience from those that know the subject material properly when creating options. Typically delicate relationships will be captured with realm-specific data.
- Validation and Understanding of Options: By understanding which options are most vital to your mode, you might be outfitted to make vital choices. Instruments for figuring out characteristic significance embrace:
- SHAP (SHapley Additive exPlanations): Serving to to quantify the contribution of every characteristic in predictions
- LIME (Native Interpretable Mannequin-agnostic Explanations): Showcasing the that means of mannequin predictions in plain language
An optimum mixture of complexity and interpretability is critical for having each good and easy to digest outcomes.
Conclusion
This brief information has addressed elementary characteristic engineering ideas, in addition to primary and superior methods, and sensible suggestions and finest practices. What many would think about a few of the most vital characteristic engineering practices — coping with lacking data, encoding of categorical knowledge, scaling knowledge, and creation of recent options — have been coated.
Function engineering is a follow that turns into higher with execution, and I hope you could have been capable of take one thing away with you which will enhance your knowledge science expertise. I encourage you to use these methods to your personal work and to be taught out of your experiences.
Do not forget that, whereas the precise share varies relying on who tells it, a majority of any machine studying mission is spent within the knowledge preparation and preprocessing part. Function engineering is part of this prolonged part, and as such must be seen with the import that it calls for. Studying to see characteristic engineering what it’s — a serving to hand within the modeling course of — ought to make it extra digestible to newcomers.
Comfortable engineering!
Matthew Mayo (@mattmayo13) holds a Grasp’s diploma in pc science and a graduate diploma in knowledge mining. As Managing Editor, Matthew goals to make advanced knowledge science ideas accessible. His skilled pursuits embrace pure language processing, machine studying algorithms, and exploring rising AI. He’s pushed by a mission to democratize data within the knowledge science group. Matthew has been coding since he was 6 years previous.