Introduction
Have you ever ever puzzled what makes some algorithms sooner and extra environment friendly than others? All of it boils down to 2 essential elements: time and house complexity. Consider time complexity because the clock ticking away, measuring how lengthy an algorithm takes to finish primarily based on the dimensions of its enter. Then again, house complexity is sort of a storage unit, retaining monitor of how a lot reminiscence the algorithm wants because the enter measurement grows. To make sense of this, we use Large O notation—a helpful option to describe the higher limits of an algorithm’s development price. Let’s dive into the fascinating world of calculating algorithm effectivity!
Overview
- Algorithms are measured by their effectivity, outlined by time and house complexity.
- Time complexity measures the execution time of an algorithm relative to enter measurement.
- House complexity tracks the reminiscence utilization of an algorithm because the enter measurement grows.
- Large O notation helps describe the higher limits of an algorithm’s development price.
- Understanding algorithm effectivity includes analyzing and optimizing each time and house complexity.
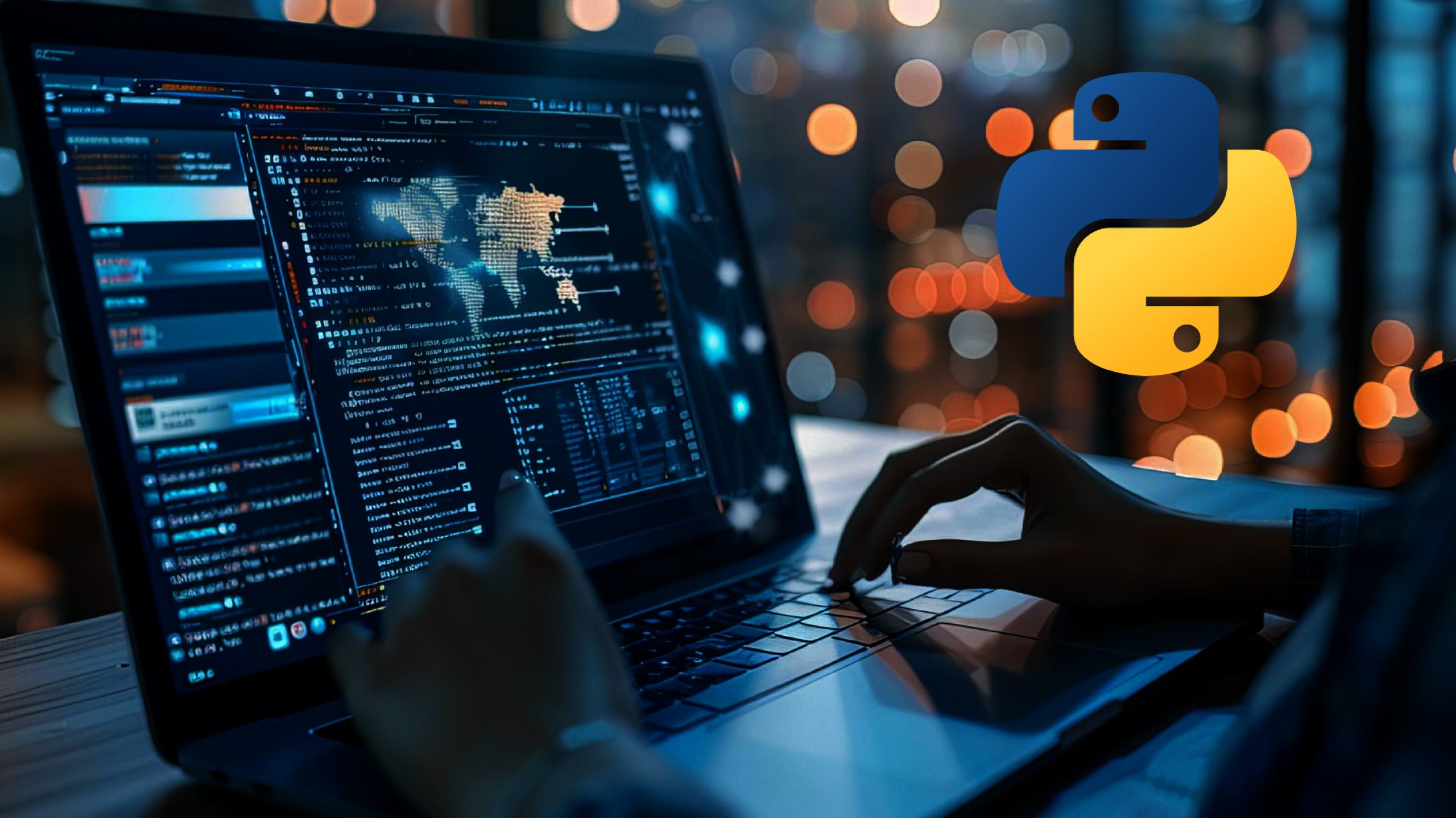
What’s Time and House Complexity?
Time complexity and house complexity are two elementary ideas used to guage the effectivity of algorithms.
Time Complexity
Time complexity refers back to the period of time an algorithm takes to finish as a perform of the enter measurement. It’s primarily a measure of the pace of an algorithm. Time complexity is often expressed utilizing Large O notation, which gives an higher sure on the algorithm’s development price. Some widespread time complexities are:
- O(1): Fixed time – The algorithm takes the identical time whatever the enter measurement.
- O(log n): Logarithmic time – The time grows logarithmically because the enter measurement will increase.
- O(n): Linear time – The time grows linearly with the enter measurement.
- O(n log n): Linearithmic time – The time grows in linear and logarithmic charges.
- O(n^2): Quadratic time – The time grows quadratically with the enter measurement.
- O(2^n): Exponential time – The time doubles with every further factor within the enter.
- O(n!): Factorial time – The time grows factorially with the enter measurement.
House Complexity
House complexity refers back to the quantity of reminiscence an algorithm makes use of as a perform of the enter measurement. It measures the effectivity of an algorithm when it comes to the quantity of reminiscence it requires to run. Much like time complexity, house complexity can be expressed utilizing Large O notation. Some widespread house complexities are:
- O(1): Fixed house – the algorithm makes use of a set quantity of reminiscence whatever the enter measurement.
- O(n): Linear house – the reminiscence utilization grows linearly with the enter measurement.
- O(n^2): Quadratic house – the reminiscence utilization grows quadratically with the enter measurement.
By analyzing each time and house complexity, you possibly can perceive an algorithm’s effectivity comprehensively and make knowledgeable choices about which algorithm to make use of for a selected drawback.
Step-by-Step Information To Calculate Algorithm Effectivity
Step 1: Perceive the Algorithm
Outline the Downside
- Clearly perceive what the algorithm is meant to do.
- Determine the enter measurement (n), sometimes the variety of components within the enter information.
Determine Primary Operations
- Decide the important thing operations within the algorithm, resembling comparisons, arithmetic operations, and assignments.
Step 2: Analyze Time Complexity
Determine Primary Operations
- Give attention to the algorithm’s most time-consuming operations, resembling comparisons, arithmetic operations, and information construction manipulations.
Rely Primary Operations
- Decide how usually every primary operation is carried out relative to the enter measurement (n).
Instance
def example_algorithm(arr):
n = len(arr)
sum = 0
for i in vary(n):
sum += arr[i]
return sum
Clarification of Code
- Initialization: sum = 0 (O(1))
- Loop: for i in vary(n) (O(n))
- Inside Loop: sum += arr[i] (O(1) per iteration, O(n) whole)
Specific Time Complexity
- Mix the operations to precise the general time complexity in Large O notation.
- Instance: The above algorithm has an O(n) time complexity.
Take into account Greatest, Common, and Worst Circumstances
- Greatest Case: The situation the place the algorithm performs the fewest steps.
- Common Case: The anticipated time complexity over all doable inputs.
- Worst Case: The situation the place the algorithm performs essentially the most steps.
Step 3: Analyze House Complexity
Determine Reminiscence Utilization
- Decide the reminiscence required for variables, information constructions, and performance name stack.
Rely Reminiscence Utilization
- Analyze the algorithm to depend the reminiscence used relative to the enter measurement (n).
Instance
def example_algorithm(arr):
n = len(arr)
sum = 0
for i in vary(n):
sum += arr[i]
return sum
House Complexity of Every Variable
- Variables: sum (O(1)), n (O(1)), arr (O(n))
Specific House Complexity
- Mix the reminiscence utilization to precise the general house complexity in Large O notation.
- Instance: The above algorithm has an O(n) house complexity.
Step 4: Simplify the Complexity Expression
Ignore Decrease-Order Phrases
- Give attention to the time period with the best development price in Large O notation.
Ignore Fixed Coefficients
- Large O notation is worried with development charges, not particular values.
Frequent Time Complexities
Time Complexity | Notation | Description |
Fixed Time | O(1) | The algorithm’s efficiency is impartial of the enter measurement. |
Logarithmic Time | O(log n) | The algorithm’s efficiency grows logarithmically with the enter measurement. |
Linear Time | O(n) | The algorithm’s efficiency grows linearly with the enter measurement. |
Log-Linear Time | O(n log n) | The algorithm’s efficiency grows in a log-linear trend. |
Quadratic Time | O(n^2) | The algorithm’s efficiency grows quadratically with the enter measurement. |
Exponential Time | O(2^n) | The algorithm’s efficiency grows exponentially with the enter measurement. |
Conclusion
Calculating the effectivity of an algorithm entails studying every time and house complexity utilizing Large O notation. Following the above talked about steps, you possibly can systematically evaluate and optimize your algorithms to make sure they perform accurately for varied enter sizes. Observe and familiarity with distinctive styles of algorithms will help you in greedy this very important factor of laptop science.
Incessantly Requested Questions
Ans. To enhance the effectivity of an algorithm:
A. Optimize the logic to cut back the variety of operations.
B. Use environment friendly information constructions.
C. Keep away from pointless computations and redundant code.
D. Implement memoization or caching the place relevant.
E. Break down the issue and resolve subproblems extra effectively.
Ans. Right here is the distinction between finest, common, and worst-case time complexities:
A. Greatest Case: The situation the place the algorithm performs the fewest steps.
B. Common Case: The anticipated time complexity over all doable inputs.
C. Worst Case: The situation the place the algorithm performs essentially the most steps.
Ans. Algorithm effectivity refers to how successfully an algorithm performs when it comes to time (how briskly it runs) and house (how a lot reminiscence it makes use of). Environment friendly algorithms resolve issues in much less time and use fewer assets.
Ans. Large O notation is a mathematical illustration used to explain the higher sure of an algorithm’s working time or house necessities within the worst-case situation. It gives an asymptotic evaluation of the algorithm’s effectivity.