Introduction
Successfully managing time is a paramount concern in programming, notably when coping with dates and occasions. Python addresses this with the Time Module, a built-in module providing an array of capabilities and strategies for seamless time-related operations. This information delves into the intricacies of the Time Module, illuminating its significance in programming and offering insights on effectively dealing with dates and occasions.Be taught fundamentals of getting present Date and Time utilizing Python
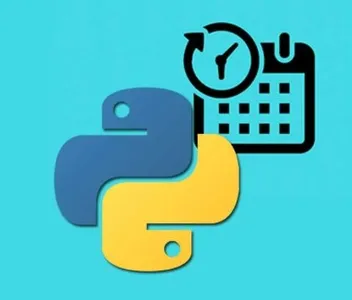
Understanding the Time Module in Python
The Time Module is an integral a part of Python, providing functionalities to work with dates, occasions, and intervals. It facilitates duties resembling measuring execution time, scheduling occasions, logging timestamps, and calculating time variations. These capabilities make it an indispensable device for time-related manipulations in programming.
Discover extra about Python Libraries for Time-Collection Evaluation
Significance of Time Administration in Programming
Exact time administration in programming holds multifaceted significance. Firstly, it ensures the effectivity and efficiency of your code. Secondly, it allows process scheduling, permitting the execution of particular operations at predetermined occasions. Lastly, efficient time administration is key for logging timestamps and computing time variations, essential facets in various purposes.
Navigating the datetime Module
Earlier than delving into the Time Module, a foundational understanding of the datetime module is essential. The datetime module furnishes lessons for manipulating dates and occasions, providing operations for creating objects, conducting arithmetic, and formatting dates and occasions.
Exploring the Time Module
Complementing the datetime module, the time module in Python focuses on time-related knowledge, represented in seconds because the epoch (January 1, 1970). It introduces capabilities resembling time(), sleep(), strftime(), strptime(), gmtime(), localtime(), mktime(), ctime(), perf_counter(), and process_time().
Evaluating datetime and time Modules
Whereas each datetime and time modules deal with dates and occasions, they serve distinct functions. The datetime module concentrates on manipulation and formatting, whereas the time module excels in time-related calculations and conversions. The selection between them hinges on particular programming wants.
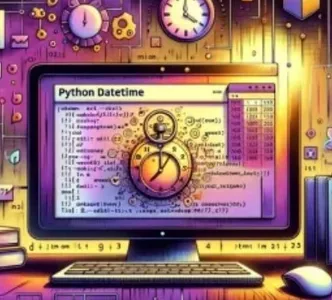
Time Module Features and Strategies
time()
Returns the present time in seconds because the epoch, helpful for measuring execution time or producing timestamps.
import time
current_time = time.time()
print("Present Time:", current_time)
sleep()
Suspends program execution for a specified period, ideally suited for introducing delays or pausing program execution.
import time
print("Begin")
time.sleep(5)
print("Finish")
strftime()
Converts a date or time object right into a string illustration primarily based on a specified format, permitting customizable output.
import time
current_time = time.localtime()
formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", current_time)
print("Formatted Time:", formatted_time)
strptime()
Converts a string illustration of a date or time right into a date or time object, serving because the reverse of strftime().
import time
date_string = "2022-01-01"
date_object = time.strptime(date_string, "%Y-%m-%d")
print("Date Object:", date_object)
gmtime()
Returns the present time in Coordinated Common Time (UTC) as a struct_time object.
import time
utc_time = time.gmtime()
print("UTC Time:", utc_time)
localtime()
Returns the present time within the native time zone as a struct_time object.
import time
local_time = time.localtime()
print("Native Time:", local_time)
mktime()
Converts a struct_time object or a tuple representing a date and time into seconds because the epoch.
import time
date_tuple = (2022, 1, 1, 0, 0, 0, 0, 0, 0)
seconds_since_epoch = time.mktime(date_tuple)
print("Seconds since Epoch:", seconds_since_epoch)
ctime()
Converts time in seconds because the epoch right into a string illustration of native time.
import time
current_time = time.time()
formatted_time = time.ctime(current_time)
print("Formatted Time:", formatted_time)
perf_counter()
Returns the worth of a efficiency counter, helpful for measuring quick durations.
import time
start_time = time.perf_counter()
# Carry out some process
end_time = time.perf_counter()
execution_time = end_time - start_time
print("Execution Time:", execution_time)
process_time()
Returns the worth of the sum of the system and consumer CPU time of the present course of, useful for measuring CPU time consumed.
import time
start_time = time.process_time()
# Carry out some process
end_time = time.process_time()
execution_time = end_time - start_time
print("Execution Time:", execution_time)
Date and Time Formatting
Formatting Directives
Formatting directives, represented by particular characters, dictate the specified format of the output when working with dates and occasions. They’re integral to the strftime() methodology.
Examples of Formatting Dates and Instances
Illustrative examples of formatting dates and occasions utilizing the strftime() methodology:
import time
current_time = time.localtime()
formatted_date = time.strftime("%Y-%m-%d", current_time)
print("Formatted Date:", formatted_date)
formatted_time = time.strftime("%H:%M:%S", current_time)
print("Formatted Time:", formatted_time)
formatted_datetime = time.strftime("%Y-%m-%d %H:%M:%S", current_time)
print("Formatted DateTime:", formatted_datetime)
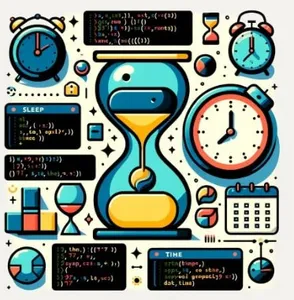
Time Zones and Daylight Saving Time
Understanding Time Zones
Time zones, demarcating areas with the identical commonplace time, are outlined by their offset from Coordinated Common Time (UTC). Greedy time zones is crucial when navigating dates and occasions throughout totally different geographical areas.
Changing Time Zones
To transform time from one zone to a different, the pytz library proves invaluable. It supplies instruments for working with time zones and executing seamless conversions.
Dealing with Daylight Saving Time
Daylight Saving Time (DST), adjusting the clock ahead by an hour in hotter months, requires consideration when working with dates and occasions. Adapting to DST ensures correct time-related outcomes.
Working with Timedelta
What’s Timedelta?
Timedelta, a category within the datetime module, represents the distinction between two dates or occasions. It facilitates arithmetic operations and time interval calculations.
Performing Arithmetic Operations with Timedelta
Timedelta permits various arithmetic operations, together with addition, subtraction, multiplication, and division.
Examples of Timedelta Utilization
Demonstrations of utilizing timedelta to calculate time intervals:
Time Module Finest Practices and Ideas
Environment friendly Time Calculations
Optimize time calculations by avoiding pointless conversions and computations, making certain code effectivity.
Error Dealing with and Exception Dealing with
Successfully deal with errors and exceptions when working with dates and occasions. Validate consumer enter, gracefully handle invalid knowledge, and make use of acceptable error-handling strategies.
Coping with Time Zone Ambiguities
Be vigilant concerning time zone ambiguities that will come up when working throughout areas. Addressing potential ambiguities ensures correct ends in your purposes.
Optimizing Efficiency
Optimize efficiency by selecting probably the most environment friendly options, resembling using the datetime module as an alternative of the time module for particular operations. Profiling your code helps establish bottlenecks for general efficiency enchancment.
Frequent Use Circumstances and Examples
Measuring Execution Time
Use the time module to measure execution time, a standard requirement in programming. Monitor the time taken by particular duties or capabilities for efficiency analysis.
Scheduling Duties
Leverage the time module to schedule duties at predefined occasions or intervals. Features like sleep() and timers facilitate the execution of duties as per your programming wants.
Logging Timestamps
Logging timestamps is significant for occasion monitoring and debugging. The time module’s capabilities and strategies supply seamless options for producing timestamps and incorporating them into your utility logs.
Calculating Time Variations
Correct time distinction calculations are integral to varied purposes. The time module, coupled with the datetime module, supplies sturdy functionalities for exact time interval and distinction calculations.
Conclusion
The Time Module in Python emerges as a potent ally for managing dates and occasions effectively. With an array of capabilities and strategies at your disposal, this module facilitates seamless time-related operations. Armed with a complete understanding of the Time Module and adherence to finest practices, you’ll be able to adeptly navigate the complexities of time in your programming endeavors, making certain accuracy and optimum efficiency.
Learn Extra about DateTime Variables in Python
Incessantly Requested Questions
A: The Time Module in Python is a built-in module that gives capabilities and strategies for dealing with dates, occasions, and time intervals. It’s essential in programming for duties resembling measuring execution time, scheduling occasions, logging timestamps, and calculating time variations, making certain environment friendly time administration in purposes.
A: Whereas each modules deal with dates and occasions, the datetime module focuses on manipulation and formatting, whereas the time module primarily offers with time-related calculations and conversions. The selection between them will depend on particular programming necessities.
A: The Time Module is usually used for measuring execution time, scheduling duties, logging timestamps, and calculating time variations. It’s versatile and relevant in various situations the place correct time administration is essential.
A: The Time Module supplies the strftime() methodology, permitting customers to format date and time objects into string representations primarily based on specified codecs. Directives like “%Y-%m-%d” and “%H:%M:%S” can be utilized for custom-made formatting.