Introduction
Pandas is a strong knowledge manipulation library in Python that gives numerous knowledge buildings, together with the DataFrame. A DataFrame is a two-dimensional labeled knowledge construction with columns of doubtless differing kinds. It’s much like a desk in a relational database or a spreadsheet in Excel. In knowledge evaluation, making a DataFrame is usually step one in working with knowledge. This text explores 10 strategies to create a Pandas DataFrame and discusses their professionals and cons.
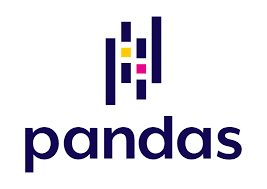
Significance of Pandas Dataframe in Knowledge Evaluation
Earlier than diving into the strategies of making a Pandas DataFrame, let’s perceive the significance of DataFrame in knowledge evaluation. A DataFrame permits us to retailer and manipulate knowledge in a structured method, making it simpler to carry out numerous knowledge evaluation duties. It offers a handy approach to set up, filter, kind, and analyze knowledge. With its wealthy set of features and strategies, Pandas DataFrame has turn into the go-to device for knowledge scientists and analysts.
Strategies to Create Pandas Dataframe
Utilizing a Dictionary
A dictionary is likely one of the easiest methods to create a DataFrame. On this methodology, every key-value pair within the dictionary represents a column within the DataFrame, the place the hot button is the column title and the worth is an inventory or array containing the column values. Right here’s an instance:
Code
import pandas as pd
knowledge = {'Identify': ['John', 'Emma', 'Michael'],
'Age': [25, 28, 32],
'Metropolis': ['New York', 'London', 'Paris']}
df = pd.DataFrame(knowledge)
Utilizing a Record of Lists
One other approach to create a DataFrame is by utilizing an inventory of lists. On this methodology, every inside checklist represents a row within the DataFrame, and the outer checklist accommodates all of the rows. Right here’s an instance:
Code
import pandas as pd
knowledge = [['John', 25, 'New York'],
['Emma', 28, 'London'],
['Michael', 32, 'Paris']]
df = pd.DataFrame(knowledge, columns=['Name', 'Age', 'City'])
Utilizing a Record of Dictionaries
One other approach to create a DataFrame is by utilizing an inventory of lists. On this methodology, every inside checklist represents a row within the DataFrame, and the outer checklist accommodates all of the rows. Right here’s an instance:
Code
import pandas as pd
knowledge = [['John', 25, 'New York'],
['Emma', 28, 'London'],
['Michael', 32, 'Paris']]
df = pd.DataFrame(knowledge, columns=['Name', 'Age', 'City'])
Whereas this methodology is straightforward and intuitive, it’s necessary to notice that utilizing an inventory of lists is probably not essentially the most memory-efficient strategy for giant datasets. The priority right here is expounded to reminiscence effectivity relatively than an absolute limitation on dataset dimension. Because the dataset grows, the reminiscence required to retailer the checklist of lists will increase, and it might turn into much less environment friendly in comparison with different strategies, particularly when coping with very massive datasets.
Issues for reminiscence effectivity turn into extra vital when working with substantial quantities of information, and various strategies like utilizing NumPy arrays or studying knowledge from exterior recordsdata could also be extra appropriate in these instances.
Utilizing a NumPy Array
You probably have knowledge saved in a NumPy array, you possibly can simply create a DataFrame from it. On this methodology, every column within the DataFrame corresponds to a column within the array. It’s necessary to notice that the instance under makes use of a 2D NumPy array, the place every row represents a file, and every column represents a characteristic.
Code
import pandas as pd
import numpy as np
knowledge = np.array([['John', 25, 'New York'],
['Emma', 28, 'London'],
['Michael', 32, 'Paris']])
df = pd.DataFrame(knowledge, columns=['Name', 'Age', 'City'])
On this instance, the array knowledge is two-dimensional, with every inside array representing a row within the DataFrame. The columns parameter is used to specify the column names for the DataFrame.
Utilizing a CSV File
Pandas offers a handy perform referred to as `read_csv()` to learn knowledge from a CSV file and create a DataFrame. This methodology is beneficial when storing a big dataset in a CSV file. Right here’s an instance:
Code
import pandas as pd
df = pd.read_csv('knowledge.csv')
Utilizing Excel Recordsdata
Like CSV recordsdata, you possibly can create a DataFrame from an Excel file utilizing the `read_excel()` perform. This methodology is beneficial when knowledge is saved in a number of sheets inside an Excel file. Right here’s an instance:
Code
import pandas as pd
df = pd.read_excel('knowledge.xlsx', sheet_name="Sheet1")
Utilizing JSON Knowledge
In case your knowledge is in JSON format, you possibly can create a DataFrame utilizing the `read_json()` perform. This methodology is especially helpful when working with net APIs that return knowledge in JSON format. Right here’s an instance:
Code
import pandas as pd
df = pd.read_json('knowledge.json')
Utilizing SQL Database
Pandas offers a strong perform referred to as `read_sql()` that means that you can create a DataFrame by executing SQL queries on a database. This methodology is beneficial when you could have knowledge saved in a relational database. Right here’s an instance:
Code
import pandas as pd
import sqlite3
conn = sqlite3.join('database.db')
question = 'SELECT * FROM desk'
df = pd.read_sql(question, conn)
Undergo the documentation: pandas.DataFrame — pandas 2.2.0 documentation
Utilizing Net Scraping
To extract knowledge from a web site, you should utilize net scraping strategies to create a DataFrame. You should use libraries like BeautifulSoup or Scrapy to scrape the information after which convert it right into a DataFrame. Right here’s an instance:
Code
import pandas as pd
import requests
from bs4 import BeautifulSoup
url="https://instance.com"
response = requests.get(url)
soup = BeautifulSoup(response.textual content, 'html.parser')
# Scrape the information and retailer it in an inventory or dictionary
df = pd.DataFrame(knowledge)
You may as well learn: The Final Information to Pandas For Knowledge Science!
Utilizing API Calls
Lastly, you possibly can create a DataFrame by making API calls to retrieve knowledge from net providers. You should use libraries like requests or urllib to make HTTP requests and retrieve the information in JSON format. Then, you possibly can convert the JSON knowledge right into a DataFrame. Right here’s an instance:
Code
import pandas as pd
import requests
url="https://api.instance.com/knowledge"
response = requests.get(url)
knowledge = response.json()
df = pd.DataFrame(knowledge)
Comparability of Completely different Strategies
Now that we’ve explored numerous strategies to create a Pandas DataFrame, let’s evaluate them based mostly on their professionals and cons.
Technique | Execs | Cons |
---|---|---|
Utilizing a Dictionary | Requires a separate file for knowledge storage. It could require further preprocessing for complicated knowledge. | Restricted management over column order. Not appropriate for giant datasets. |
Utilizing a Record of Lists | Easy and intuitive. Permits management over column order. | Requires specifying column names individually. Not appropriate for giant datasets. |
Utilizing a Record of Dictionaries | Offers flexibility in specifying column names and values. Permits management over column order. | Requires extra effort to create the preliminary knowledge construction. Not appropriate for giant datasets. |
Utilizing a NumPy Array | Environment friendly for giant datasets. Permits management over column order. | Requires changing knowledge right into a NumPy array. Not appropriate for complicated knowledge buildings. |
Utilizing a CSV File | Appropriate for giant datasets. Helps numerous knowledge sorts and codecs. | Requires a separate file for knowledge storage. Could require further preprocessing for complicated knowledge. |
Utilizing Excel Recordsdata | Helps a number of sheets and codecs. Offers a well-recognized interface for Excel customers. | Requires knowledge to be in JSON format. It could require further preprocessing for complicated knowledge. |
Utilizing JSON Knowledge | Appropriate for net API integration. Helps complicated nested knowledge buildings. | Requires knowledge to be in JSON format. Could require further preprocessing for complicated knowledge. |
Utilizing SQL Database | Appropriate for giant and structured datasets. Permits complicated querying and knowledge manipulation. | Requires a connection to a database. Could have a studying curve for SQL queries. |
Utilizing Net Scraping | Permits knowledge extraction from web sites. Can deal with dynamic and altering knowledge. | Requires data of net scraping strategies. Could also be topic to web site restrictions and authorized issues. |
Utilizing API Calls | Permits integration with net providers. Offers real-time knowledge retrieval. | Requires data of API authentication and endpoints. Could have limitations on knowledge entry and charge limits. |
You may as well learn: A Easy Information to Pandas Dataframe Operations
Conclusion
On this article, we explored totally different strategies to create a Pandas DataFrame. We mentioned numerous strategies, together with utilizing dictionaries, lists, NumPy arrays, CSV recordsdata, Excel recordsdata, JSON knowledge, SQL databases, net scraping, and API calls. Every methodology has its personal professionals and cons, and the selection will depend on the precise necessities and constraints of the information evaluation activity. Moreover, we discovered about further strategies supplied by Pandas, such because the read_csv(), read_excel(), read_json(), read_sql(), and read_html() features. By understanding these strategies and strategies, you possibly can successfully create and manipulate DataFrames in Pandas to your knowledge evaluation initiatives.